]
DESCRIPTION
This command generates an Yii Web Application
at the specified location.
- app-path: required, the directory for the new app
- vcs: optional, version control system you're going to use
```
For example:
```
cd /path/to/yii-1.1.16.bca042/framework/
yiic webapp /path/to/my/new/app git
```
]
---
.left-column[
## Getting started
### - Creating a new project
### - Directory structure
]
.right-column[
```
index.php Web application entry script file
index-test.php entry script file for the functional tests
assets/ published resource files
css/ CSS files
images/ image files
themes/ application themes
protected/ protected application files
yiic yiic command line script for Unix/Linux
yiic.bat yiic command line script for Windows
yiic.php yiic command line PHP script
commands/ customized 'yiic' commands
components/ reusable user components
config/ configuration files
controllers/ controller class files
data/ the sample database
extensions/ third-party extensions
messages/ translated messages
models/ model class files
runtime/ temporarily generated files
tests/ test scripts
views/ controller view and layout files
layouts/ layout view files
site/ view files for the 'site' controller
```
]
---
.left-column[
## Getting started
### - Creating a new project
### - Directory structure
### - Modules
]
.right-column[
## What's a Module?
- self-contained software unit that consists of models, views, controllers and other supporting components
- must reside inside of an application
- for a large-scale application, we may divide it into several modules
- commonly used features, may be developed in terms of modules so that they can be reused
## How to use Modules?
Faster way to create one is with [Gii](http://www.yiiframework.com/doc/guide/1.1/en/topics.gii) code generation tool.
Once created simply add it to your config file:
```php
return array(
......
'modules'=>array('my_new_module',...),
......
);
```
]
---
.left-column[
## Getting started
### - Creating a new project
### - Directory structure
### - Modules
### - Config & autoload
]
.right-column[
Based on arrays. Initial config files after creating app:
```
console.php console applications use separate config file
database.php the database configuration
main.php the main configuration file
test.php the configuration for the functional tests
```
Basically you can put almost all the configurations in the `main.php` but often good practices are to:
- Split into multiple configuration files for better management. Example including separate config file from `main.php`:
```php
'db'=>require(dirname(__FILE__).'/database.php'),
```
- Reutilize between console and web apps.
- Create custom config files excluded from version control that will allow each environment different configurations.
- Merge configuration files:
```php
if (file_exists(dirname(__FILE__) . '/custom.params.php')) {
$params = CMap::mergeArray($params, require(dirname(__FILE__) . '/custom.params.php'));
}
....
'params' => $params,
```
Learn more details about everything that can be configured in [this wiki](http://www.yiiframework.com/wiki/59/).
]
---
.left-column[
## Handling requests
### - Controllers and actions
]
.right-column[
Simply create your controllers as the following example:
```php
class SiteController extends CController
{
public function actionIndex()
{
// ...
}
public function actionContact()
{
// ...
}
}
```
And then you'll be able to access each of the actions like this:
```
http://hostname/index.php?r=site/index
http://hostname/index.php?r=site/contact
```
]
---
.left-column[
## Handling requests
### - Controllers and actions
### - URL management
]
.right-column[
```php
// From controller
$this->createUrl($route,$params);
$this->createUrl('site/contact',array(
'department'=>'support'
));
// From anywhere
Yii::app()->createUrl($route,$params);
// Absolute
Yii::app()->createAbsoluteUrl($route,$params);
// Create link from view (single array)
CHtml::link('Edit post', array('/post/edit','id'=>$post->id));
// Other way to create url from view
$url=CHtml::normalizeUrl(array('/post/edit','id'=>$post->id));
```
You can use this [Yii-JSUrlManager](https://github.com/ains/Yii-JSUrlManager) extension and then run from JS: `Yii.app.createUrl('site/contact',{'department':'support'});`
To achieve [friendly URLs](http://www.yiiframework.com/doc/guide/1.1/en/topics.url#user-friendly-urls) and more go to `urlManager` config section and adjust how you want your urls to behave and define all the url rules/patterns.
```php
'rules'=>array(
// the url you want => module/controller/action
'get-in-touch'=>'site/contact',
),
```
]
---
.left-column[
## Handling requests
### - Controllers and actions
### - URL management
### - Filters
]
.right-column[
- Executed before and/or after a controller action executes
- For example, the [access control filter](http://www.yiiframework.com/doc/guide/1.1/en/topics.auth#access-control-filter)
- Can be defined
- As methods of the controller
- As individual classes
Example of filter and activating it in the controller:
```php
File: ApiController.php
...
public function filters() {
return array(
'userAgent',
);
}
...
public function filterUserAgent($filterChain) {
$userAgent=Yii::app()->request->userAgent;
if (empty($userAgent)) {
throw new CHttpException(403, 'No User-Agent provided');
}
$filterChain->run();
}
```
]
---
.left-column[
## Handling requests
### - Controllers and actions
### - URL management
### - Filters
### - Query and Post params
]
.right-column[
There are many ways you can access `$_GET` and `$_POST` data, let's see some:
```php
// Plain old not-so-safe ways
$page=$_GET['page'];
$email=$_POST['email'];
// Yii way
// Get query
Yii::app()->request->getQuery('param_name');
// Get post
Yii::app()->request->getPost('param_name');
// Get or post
Yii::app()->request->getParam('param_name');
// Action Parameter Binding
class PostController extends CController
{
public function actionCreate($category, $language='en')
{
// ...
}
}
```
]
---
.left-column[
## Views and assets
### - Views
]
.right-column[
Views are the presentation / HTML part of your application.
```php
// Parts that are common for the whole app
protected/views/layouts/main.php
// The view for the contact form action
protected/views/site/contact.php
// Partial view to be included in contact.php
// after sending the form
protected/views/site/_thanks.php
```
Rendering views:
```php
// Layouts are automatically rendered because
// normal controller/action views extend from them
// Rendering a view from controller
File: SiteController.php
public function actionContact() {
$this->render('contact', $params);
}
// Partial views don't extend the layout
// and are called like this
$this->renderPartial('_thanks.php', $params);
```
]
---
.left-column[
## Views and assets
### - Views
### - Themes
]
.right-column[
Yii supports multiple visual themes for your application.
To create a theme simply create the folder and place the views inside
as you are working with the normal `protected/views` directory:
`webroot/themes/my_new_theme`
To use it set it in your config file:
```php
'theme'=>'my_new_theme',
```
Your theme can even be empty, because views have fallback to the main views
directory of the application.
More on theming in [the guide](http://www.yiiframework.com/doc/guide/1.1/en/topics.theming).
]
---
.left-column[
## Views and assets
### - Views
### - Themes
### - Assets and scripts
]
.right-column[
Loading assets and registering scripts:
```php
// Using client script manager
$clientScript=Yii::app()->clientScript;
// Register css / js files
$clientScript->registerCssFile(
Yii::app()->baseUrl.'/css/style.css');
$clientScript->registerScriptFile(
Yii::app()->baseUrl.'/js/scripts.js');
// Register a script block
$id='my_alert';
$script="alert('test');";
$position=CClientScript::POS_READY; // jQuery ready
$clientScript->registerScript($id, $script, $position);
```
You can also use the Asset manager for publishing assets:
```php
Yii::app()->getAssetManager()->publish(
Yii::getPathOfAlias('webroot')."/img/picture.jpg");
```
To minify/unify assets for performance you can either map them to a single using
Yii built in functionality or you can use a extension such as [minScript](http://www.yiiframework.com/extension/minscript).
]
---
.left-column[
## Database
### - Setup
]
.right-column[
First create your database tables from your favorite app. PhpMyAdmin, Toad, HeidiSQL.
Then configure the application to use your DB:
```php
// File: protected/config/database.php
// This is the database connection configuration.
return array(
'connectionString' => 'mysql:host=localhost;dbname=test',
'emulatePrepare' => true,
'username' => 'root',
'password' => '',
'charset' => 'utf8'
);
```
Now you could generate all the AR models with Gii code generation tool, but we'll see that later!
]
---
.left-column[
## Database
### - Setup
### - ActiveRecord
]
.right-column[
```php
// Create
$post=new Post;
$post->title='sample post';
$post->content='post body content';
$post->save();
// find the first row satisfying the specified condition
$post=Post::model()->find($condition,$params);
// find the row with the specified primary key
$post=Post::model()->findByPk($postID,$condition,$params);
// find the row with the specified attribute values
$post=Post::model()->findByAttributes($attributes);
// find the first row using the specified SQL statement
$post=Post::model()->findBySql($sql,$params);
// find all rows satisfying the specified condition
$posts=Post::model()->findAll($condition,$params);
// assuming there is a post whose ID is 10
$post=Post::model()->findByPk(10);
// delete the row from the database table
$post->delete();
```
]
---
.left-column[
## Database
### - Setup
### - ActiveRecord
### - DataProvider, Sort, paginate
]
.right-column[
```php
$criteria = new CDbCriteria;
$criteria->compare('id', $this->id);
$criteria->compare('date_add', $this->date_add, true);
$criteria->compare('date_upd', $this->date_upd, true);
return new CActiveDataProvider('User', array(
'criteria' => $criteria,
));
```
CActiveDataProvider comes already with [Pagination](http://www.yiiframework.com/doc/api/1.1/CPagination) and [Sort](http://www.yiiframework.com/doc/api/1.1/CSort) but you can use those
components individually from a controller.
]
---
.left-column[
## Database
### - Setup
### - ActiveRecord
### - DataProvider, Sort, paginate
### - DAO/SQL
]
.right-column[
```php
$connection=Yii::app()->db; // assuming you have configured a "db" connection
$sql="SELECT * FROM USERS";
$command=$connection->createCommand($sql);
$rowCount=$command->execute(); // execute the non-query SQL
$dataReader=$command->query(); // execute a query SQL
$rows=$command->queryAll(); // query and return all rows of result
$row=$command->queryRow(); // query and return the first row of result
$column=$command->queryColumn(); // query and return the first column of result
$value=$command->queryScalar(); // query and return the first field in the first row
```
]
---
.left-column[
## Database
### - Setup
### - ActiveRecord
### - DataProvider, Sort, paginate
### - DAO/SQL
### - Good practices
]
.right-column[
Always work in such way that you bind parameters and never allow direct user input data to
be used in a SQL call, this way you prevent SQL injections.
]
---
.left-column[
## Database
### - Setup
### - ActiveRecord
### - DataProvider, Sort, paginate
### - DAO/SQL
### - Good practices
### - Migrations
]
.right-column[
- Use `yiic migrate` command line tool to create migration files or to apply new migrations
- Migrations can be written either in plain SQL or OOP
]
---
.left-column[
## Forms
### - FormModel & ActiveRecord
]
.right-column[
Both FormModel and ActiveModel extend from a parent Model class so they work in a very similar way.
```php
// FormModel
class ContactForm extends CFormModel
{
public $name;
...
public function rules()
{
...
}
public function attributeLabels()
{
...
}
}
// ActiveRecord
class ApiKey extends CActiveRecord {
public function tableName() {
return 'api_key';
}
}
```
]
---
.left-column[
## Forms
### - FormModel & ActiveRecord
### - Validating data
]
.right-column[
```php
// File: ContactForm.php
// Define validation rules of the model.
public function rules()
{
return array(
array('name, email, subject, body', 'required'),
array('email', 'email'),
...
);
}
```
See here all the available [validators](http://www.yiiframework.com/doc/guide/1.1/en/form.model#declaring-validation-rules).
Not validated fields must have a `safe` validation rule.
New custom validator can be easily created as a method inside the model and use use it in the rules.
Rules can also apply only to certain validation scenario, by using `on`.
]
---
.left-column[
## Forms
### - FormModel & ActiveRecord
### - Validating data
### - View of the form
]
.right-column[
```php
beginWidget('CActiveForm', array(
'id'=>'contact-form',
'enableClientValidation'=>true,
'clientOptions'=>array(
'validateOnSubmit'=>true,
),
)); ?>
errorSummary($model); ?>
labelEx($model,'name'); ?>
textField($model,'name'); ?>
error($model,'name'); ?>
labelEx($model,'email'); ?>
textField($model,'email'); ?>
error($model,'email'); ?>
endWidget(); ?>
```
]
---
.left-column[
## Forms
### - FormModel & ActiveRecord
### - Validating data
### - View of the form
### - Processing form
]
.right-column[
```php
public function actionContact()
{
$model=new ContactForm;
if(isset($_POST['ContactForm']))
{
$model->attributes=$_POST['ContactForm'];
if($model->validate())
{
... send mail here ..
Yii::app()->user->setFlash('contact',
'Thank you for contacting us.');
$this->refresh();
}
}
$this->render('contact',array('model'=>$model));
}
```
]
---
layout: false
.left-column[
## Code Generation
]
.right-column[
## Gii: Automatic Code Generation tool
A module that provides a web interface to:
- Generate Models from database tables
- Generate CRUD: create, read, update, delete
- Generate Module structure
It's easy to use. Just [enable it](http://www.yiiframework.com/doc/guide/1.1/en/topics.gii#using-gii) in the config file and browse to `http://hostname/path/to/index.php?r=gii`.
The generator tool can also be customized, templated, and extended.
]
---
.left-column[
## Widgets
### - What's a widget?
]
.right-column[
Widgets are small components of your application that are mainly used from views.
The most direct example could be the [CMenu](http://www.yiiframework.com/doc/api/1.1/CMenu/) widget that allows you to
insert a navigation menu in your application by providing an array of items in the
menu. All the logic of detecting active item, generating all html, and so on would
be taken care from the widget.
```php
// Running a widget from a view
beginWidget('path.to.WidgetClass'); ?>
...body content that may be captured by the widget...
endWidget(); ?>
// or
widget('path.to.WidgetClass'); ?>
```
]
---
.left-column[
## Widgets
### - What's a widget?
### - Example of widgets
]
.right-column[
- Paginator, Grid, ActiveForm, Menu, Breadcrumbs
- Yii comes with most of jQuery UI components already defined as widgets: Autocomplete, Accordion, Dialog, Datepicker and so on
- Make your own!
]
---
.left-column[
## Widgets
### - What's a widget?
### - Example of widgets
### - Creating widgets
]
.right-column[
Create your new widget and its view:
```php
// File: protected/widgets/MyWidget.php
number;
$this->render('myWidget', array('var'=>$var));
}
}
// File: protected/widgets/views/myWidget.php
widget('application.widgets.MyWidget',
array('number'=>2)); ?>
```
]
---
.left-column[
## User session and security
### - Log in
]
.right-column[
```php
public function actionLogin()
{
$model=new LoginForm;
if(isset($_POST['LoginForm']))
{
$model->attributes=$_POST['LoginForm'];
if($model->validate() && $model->login())
$this->redirect(Yii::app()->user->returnUrl);
}
$this->render('login',array('model'=>$model));
}
// LoginForm.php uses components/UserIdentity.php
public function login()
{
if($this->_identity===null)
{
$this->_identity=new UserIdentity(
$this->username,
$this->password);
$this->_identity->authenticate();
}
if($this->_identity->errorCode===UserIdentity::ERROR_NONE)
{
$duration=$this->rememberMe ? 3600*24*30 : 0;
Yii::app()->user->login($this->_identity,$duration);
return true;
}
else
return false;
}
```
]
---
.left-column[
## User session and security
### - Log in
### - Get the user
]
.right-column[
```php
// Check if user is logged in
var_dump(Yii::app()->user->isGuest);
// Get current user id
Yii::app()->user->id;
// Set / get session state
Yii::app()->user->setState("login_terms_accepted", true);
Yii::app()->user->getState("login_terms_accepted");
// Set / get session flash message
Yii::app()->user->setFlash("contact", "Email sent!");
if (Yii::app()->user->hasFlash("contact")) {
echo Yii::app()->user->getFlash("contact");
}
```
User component can be extended by creating a `WebUser` component in `protected/components/WebUser.php` that extends `CWebUser` and change the config to start using it.
You can also work directly with the `Yii::app()->session` managing the session data, for details look at [CHttpSession](http://www.yiiframework.com/doc/api/1.1/CHttpSession).
]
---
.left-column[
## User session and security
### - Log in
### - Get the user
### - Cookies
]
.right-column[
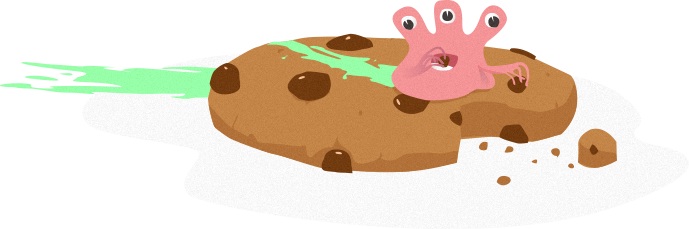
### HEAVY JELLY LOVES COOKIES
```php
// Read a cookie
$value = '';
if (isset(Yii::app()->request->cookies['cookie_name'])) {
$value = Yii::app()->request->cookies['cookie_name']->value;
}
// Write a cookie (IMPORTANT: Reload required)
$cookie=new CHttpCookie('name', 'value', $options);
Yii::app()->request->cookies['cookie_name'] = $cookie;
// Deleting a cookie
unset(Yii::app()->request->cookies['cookie_name']);
```
]
---
.left-column[
## User session and security
### - Log in
### - Get the user
### - Cookies
### - Access control & RBAC
]
.right-column[
```php
// Inside controller
public function filters() {
return array(
'accessControl', // perform access control
);
}
public function accessRules() {
return array(
array('allow',
// allow all logged user access contact action
'actions' => array('contact'),
'users' => array('@'),
),
// allow role manager to access all the actions
array('allow',
'users' => array('@'),
'roles' => array("manager"),
),
// deny access to rest of users
array('deny',
'users' => array('*'),
),
);
}
```
Go ahead and learn more about the [Access Control Filter](http://www.yiiframework.com/doc/guide/1.1/en/topics.auth#access-control-filter) and the [Role-Based Access Control](http://www.yiiframework.com/doc/guide/1.1/en/topics.auth#role-based-access-control) system.
]
---
.left-column[
## User session and security
### - Log in
### - Get the user
### - Cookies
### - Access control & RBAC
### - Security
]
.right-column[
- Cross-site Scripting Prevention (CHtmlPurifier)
- Cross-site Request Forgery Prevention (CSRF token)
- Cookie Attack Prevention (enableCookieValidation)
]
---
layout: false
.left-column[
## I18N
]
.right-column[
## Internationalization
Enable multiple languages:
```php
// In protected/config/main.php
'sourceLanguage'=>'en',
'language'=>'es',
// Then in controller change as required
Yii::app()->language='it';
```
Translate strings:
```php
Yii::t('main', 'The user "{username}" does not exist.',
array('{username}'=>$username));
// translations is saved in arrays
// protected/messages/es/main.php
'The user "{username}" does not exist.'=>'El usuario "{username}" no existe.',
// protected/messages/en/main.php
// protected/messages/it/main.php
```
Massively extract and manage translatios using `yiic message` tool.
Localization can also be done for [Date, Time and Numbers](http://www.yiiframework.com/doc/guide/1.1/es/topics.i18n#date-and-time-formatting).
]
---
layout: false
.left-column[
## Console
]
.right-column[
## Command line tools
You can use existing command line tools or create your own.
Go to your project's `protected` folder and run `yiic`:
```
-bash-4.2$ php yiic.php
Yii command runner (based on Yii v1.1.16)
Usage: yiic.php [parameters...]
The following commands are available:
- message
- migrate
- shell
- webapp
To see individual command help, use the following:
yiic.php help
```
Example of [creating a new custom command](http://www.yiiframework.com/doc/guide/1.1/en/topics.console#creating-commands):
`protected/commands/EmailSenderCommand.php`
]
---
layout: false
.left-column[
## Debugging and Logging
]
.right-column[
## Debugging
```php
// php
print_r();
var_dump();
die();
// yii
CVarDumper::dump($var,$depth=10,$highlight=false);
Yii::app()->end();
```
## Logging
```php
// logging a message of type:
// trace, warning, error, info, profile
Yii::log($msg,$level=CLogger::LEVEL_INFO,$category);
// by default logs are written to
// protected/runtime/application.log
// logging behavior can be adjusted from the log component
// in the config file, and you can define multiple log
// routes such as file, db, email
// and configure what levels and categories to log
```
]
---
.left-column[
## Performance
### - Performance Tuning
]
.right-column[
The following check list should be followed in order to improve the performance of the application:
- Enabling APC Extension
- Disabling Debug Mode
- Using yiilite.php
- Using Caching Techniques
- Database Optimization
- Minimizing Script Files and Symlinking assets
]
---
.left-column[
## Performance
### - Performance Tuning
### - Caching
]
.right-column[
Yii supports multiple ways of caching data and multiple caching technologies.
- You can cache Data, Fragment (views), full pages, and also dynamic caching, cache dependencies
- You can use simple file cache, apc, mem, xcache, db cache and many more
Simply configure the cache component of your application and start [using it](http://www.yiiframework.com/doc/guide/1.1/en/caching.overview).
```php
// Config
array(
'components'=>array(
'cache'=>array(
'class'=>'system.caching.CFileCache',
),
),
);
// Use
$value=Yii::app()->cache->get($id);
if($value===false)
{
// regenerate $value because it is not found in cache
// and save it in cache for later use:
// Yii::app()->cache->set($id,$value);
}
```
]
---
.left-column[
## Special topics
### - Helpers
]
.right-column[
Helper classes:
- in views we often need some code snippets to do tiny tasks such as formatting data or generating HTML tags
- place all of these code snippets in a view helper class
- use the helper class in your view files
- Yii has a powerful [CHtml](http://www.yiiframework.com/doc/api/1.1/CHtml) helper that is used a lot in forms and not only
- other interesting helpers to use from any part of the application are [CJSON](http://www.yiiframework.com/doc/api/1.1/CJSON) and [CJavaScript](http://www.yiiframework.com/doc/api/1.1/CJavaScript) that allow you for example to manage json and/or escape javascript data
]
---
.left-column[
## Special topics
### - Helpers
### - Components
]
.right-column[
Yii comes with a lot of default components attached to the application but you
can also create new ones, either to extend current ones or to create your own.
```php
// File: protected/components/SMSComponent.php
class SMSComponent extends CComponent
{
public $my_phone;
public function sendSMS($to)
{
...
}
}
// Configure the app to load it
// application components
'components'=>array(
'sms'=>array(
'class' => 'application.components.SMSComponent',
'my_phone' => 123456,
),
)
// Use the component from anywhere
$to=5554444;
Yii:app()->sms->sendSMS($to);
```
]
---
.left-column[
## Special topics
### - Helpers
### - Components
### - Behavior
]
.right-column[
Behaviors can be used for some of the following reasons:
- Allow possibility of a class to extend multiple classes methods, basically creating a Behavior with 2 methods and attaching it to an object, that object will have the 2 methods too
- Other reason is to be able to make an object behave as you want, and reutilize that, for example:
- [Timestamp](http://www.yiiframework.com/doc/api/1.1/CTimestampBehavior) behavior
- Slug behavior
- Etc
]
---
.left-column[
## Special topics
### - Helpers
### - Components
### - Behavior
### - Events
]
.right-column[
What is an event? It is the fact that we want to express that in certain points in our source code something happens!
More info on events in this [wiki](http://www.yiiframework.com/wiki/327/events-explained/) and in the [guide](http://www.yiiframework.com/doc/guide/1.1/en/basics.component#component-event).
]
---
.left-column[
## Special topics
### - Helpers
### - Components
### - Behavior
### - Events
### - Webservices and APIs
]
.right-column[
Yii comes with an prebuilt and automated way to create [SOAP webservices](http://www.yiiframework.com/doc/guide/1.1/en/topics.webservice).
As for REST API, with some controllers, url routing and some imagination you can build a REST API in a few steps.
]
---
.left-column[
## Special topics
### - Helpers
### - Components
### - Behavior
### - Events
### - Webservices and APIs
### - Testing
]
.right-column[
Yii supports TDD, Unit Testing, and Functional testing.
More on testing in the [guide](http://www.yiiframework.com/doc/guide/1.1/en/test.overview).
]
---
.left-column[
## Extending Yii
]
.right-column[
## Extending and customizing Yii
Yii is the most flexible and customizable thing on the planet, so.. please dare and push its boundaries!
Take a step further than Yii. Don't just use its widgets and tools, create custom tools and build great original products!
- What resources are out there?
- There are [2k extensions](http://www.yiiframework.com/extensions/) published on the site for you to use them
- What can I extend and customize? [Almost everything](http://www.yiiframework.com/doc/guide/1.1/en/extension.overview)
- Components: user, urlManager and rules, db, logging, cache
- Widgets: Grid, ActiveForm, Pagination, Breadcrumbs, Menu
- Helpers: CHtml, make your own?
- And the list is endless..
- It's okay to use all its shortcuts to get stuff done faster but building a great application means to customize the user interface and user experience
]
---
class: center, middle
## Some good practices
---
.left-column[
## Some good practices
]
.right-column[
- Set framework path inside a configuration file
- Bigger application, use modules and better organization of files
- For god sake, clean generated code! clean unused code!
- Multiple bootstrap index.php files with different config
- Local virtual hosts
- Save private data / uploads in protected folder
- Use config file for auth_tasks and rebuild it by command-line for RBAC
- Extend Procedures Migrations if you DB uses migrations
- Proper AJAX Requests and working with Urls and jQuery widgets
- BAD! Don't use AR's search() metod to find sigle item, it's purpose is to be used in grids and lists
- As general developer: Good IDE(NetBeans/Phpstorm), CVS(GIT), API & DOCS - RTFM!!
- Read Yii's [conventions](http://www.yiiframework.com/doc/guide/1.1/en/basics.convention) too on how to organize and name stuff and [MVC best practices](http://www.yiiframework.com/doc/guide/1.1/en/basics.best-practices)
]
---
name: comparing
class: center, middle
## Comparing Yii 1 with Yii 2
---
.left-column[
## Yii 1 vs Yii 2
]
.right-column[
What changes.. basically, more to do and also cool stuff but let's see:
- Use of composer
- PHP 5.4
- Namespaces / Use
- Short arrays `[]`
- Yii 1 was jQuery, Yii 2 is Bootstrap
- Simple or advanced app structure
- Advanced app basically is multi-app with common shared libraries
- Index.php file now is in `web` public directory
- In advanced multi-apps each one must have a VHOST, frontend, backend, api, etc
- Environment files and more to configure
- Goodies official extensions maintained by Yii core team, mongo, redis, swift, twig, among others
- In View `$this` is a `Yii\web\view` object not a controller, to get the controller must do `$this->context`
- Console apps commands are controllers now
For more info see [Upgrading from Version 1.1](http://www.yiiframework.com/doc-2.0/guide-intro-upgrade-from-v1.html).
]
---
class: center, middle
## Final conclusions
---
.left-column[
## Final conclusions
### - Example projects
]
.right-column[
Listing some projects that have been done by HeavyDots using Yii:
Saas / CRM
https://nexocrm.com/ Yii 1 app, Yii 2 website
Social networks
http://mitibox.heavydots.com/ Yii 1
http://branbee.heavydots.com/ Yii 1
Websites
http://heavydots.com/ Yii 2
]
---
.left-column[
## Final conclusions
### - Example projects
### - Links
]
.right-column[
Guide & API
http://www.yiiframework.com/doc/guide/
http://www.yiiframework.com/doc/api/
Extensions, wiki and other tutorials
http://www.yiiframework.com/extensions/
http://www.yiiframework.com/demos/
http://www.yiiframework.com/download/
http://www.yiiframework.com/wiki/
http://www.yiiframework.com/tutorials/
http://www.yiiframework.com/doc/blog/
Github
https://github.com/yiisoft
News
http://yiifeed.com/
Social networks
https://www.facebook.com/groups/yiitalk/
https://twitter.com/yiiframework
]
---
class: center, middle
## Questions?
---
name: last-page
class: center, middle
template: inverse
## Thank you..
..for watching!
..Yii team & community
..[BCN PHP Meetup](https://www.meetup.com/es-ES/php-377/) and [Ironhack](https://www.meetup.com/es-ES/ironhack-barcelona/) for organizing the event!
..[remark](http://github.com/gnab/remark) presentation tool!
..Jorge, Richard & Angel for feedback!
..Pablo for feedback and illustration!
---
class: center, middle
## Ready to hack?
---
class: center, middle